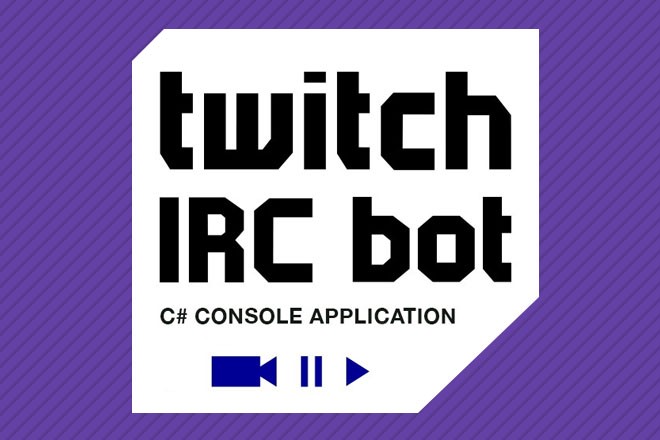
Background
Video game streaming has been an important part of the gaming community since Twitch took in its first breath of life in 2011. Gamers like to watch other people play games for information and entertainment. However, live streams take this concept one step further. These streams allow the audience or viewers to ask questions about the game being streamed and to interact with the streamer or broadcaster in real-time.
There are many different aspects to streaming, ranging from foreground content (game and broadcaster input) to background content (music and chat bots). Chat bots, in particular, help not only the broadcaster interact with the audience, but also entertain them when the broadcaster is too busy to respond. Coming from personal experience as a broadcaster, many of my viewers come to my streams not for the foreground content, but for my custom chat bot and myself. My personal advice is that anyone can watch gameplay videos, but personality and uniqueness bring your audience back.
This communication between the audience and the broadcaster is driven by an Internet Relay Chat (IRC) client/server relationship that allows users to communicate together via text. This post goes over the general structure of Twitch chat bots without a graphical user interface (GUI).
Bot requirements
Before working with this IRC Console Application, you need 3 things:
- A broadcaster’s username
- Your bot’s name from your second Twitch account
- An OAuth password to allow the bot to enter the broadcaster’s channel
- Under your main account, you need to have a Client ID for this application
- This can be obtained by registering your application under the “Connections” tab within the “Settings” menu of Twitch and grabbing it there
- Go to Twitch Chat OAuth Password Generator as your bot account to generate this password
- If you need additional info, please check out the official authentication documentation
Getting started
Make a new console application solution. My namespace is TwitchBotDemo if you want to follow exactly what I have written. You need three classes: Program.cs, IrcClient.cs, and PingSender.cs. Replace the generated code inside these class files with the code below.
For demo purposes, I have implemented the bot settings inside Program.cs as private static string variables. Please replace the values within these variables with the appropriate info you’ve gathered from the requirements explained above.
And that’s it! You should be able to build and run this application in one fell swoop.
Code
C# - Program.cs
C# - ircclient.cs
C# - pingsender.cs
Notes
I’ve used Visual Studio 2015 Community with .NET Framework 4.6.1 to make this demonstration. I have also used a YouTube chat bot tutorial for the IrcClient code (referenced inside the IrcClient.cs) because it is short, sweet and straight to the point. I followed this tutorial for my first Twitch bot until I needed more commands and a way to keep my bot’s connection alive.
Closing
I hope you have a basic understanding of how Twitch IRC bots work and a foundation to expand this logic. If you enjoyed this article and want to get in contact with me with more questions about this bot or would be interested in my Twitch streams, please check out my GitHub or Twitch channel.